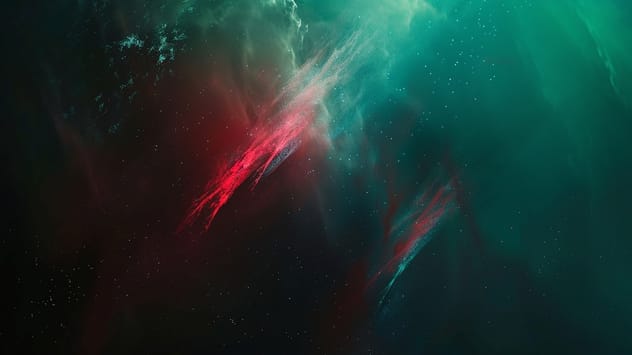
Bot spoofing and how to detect it with Arcjet
We're adding more detailed verification options for developers where every request will be checked behind the scenes using published IP and reverse DNS data for common bots.
Arcjet changelog of product updates for April 2024.
Arcjet helps developers protect their apps in just a few lines of code. Implement rate limiting, bot protection, email verification & defend against common attacks.
This is the changelog of product updates for April 2024.
We released 2 new JS SDK versions in April: v1.0.0-alpha.11 and v1.0.0-alpha.12
Every call to protect
in the Arcjet SDK now returns IP address metadata about the IP location, the IP network details (AS) and the type of IP e.g. VPN or proxy.
Field availability depends on the data we have, but geolocation and network data is available for all IPs.
import arcjet, { shield } from "@arcjet/next";
import { NextResponse } from "next/server";
const aj = arcjet({
key: process.env.ARCJET_KEY,
rules: [
// Protect against common attacks with Arcjet Shield
shield({
mode: "LIVE", // will block requests. Use "DRY_RUN" to log only
}),
],
});
export async function POST(req: Request) {
const decision = await aj.protect(req);
if (decision.ip.hasCountry()) {
return NextResponse.json({
message: `Hello ${decision.ip.countryName}!`,
country: decision.ip,
});
}
if (decision.isDenied()) {
return NextResponse.json({ error: "Forbidden" }, { status: 403 });
}
return NextResponse.json({
message: "Hello world",
});
}
A simple Next.js POST route handler returning IP country data.
For the IP address 8.8.8.8
you might get the following response:
{
"name": "Hello United States!",
"ip": {
"country": "US",
"countryName": "United States",
"continent": "NA",
"continentName": "North America",
"asn": "AS15169",
"asnName": "Google LLC",
"asnDomain": "google.com"
}
}
Full details and all fields are listed in our docs (Next.js and Node.js).
Arcjet Shield protects your application against common attacks, including the OWASP Top 10. This was previously always enabled, but it can now be configured.
We still add the rule by default, but this is now deprecated and a warning will be logged to remind you. If you want to continue using Shield in future then you'll need to specifically add the rule.
import arcjet, { shield } from "@arcjet/node";
import http from "node:http";
const aj = arcjet({
// Get your site key from https://app.arcjet.com
// and set it as an environment variable rather than hard coding.
key: process.env.ARCJET_KEY,
rules: [
// Protect against common attacks with Arcjet Shield
shield({
mode: "DRY_RUN", // Change to "LIVE" to block requests
}),
],
});
const server = http.createServer(async function (
req: http.IncomingMessage,
res: http.ServerResponse,
) {
const decision = await aj.protect(req);
for (const result of decision.results) {
console.log("Rule Result", result);
}
console.log("Conclusion", decision.conclusion);
if (decision.isDenied()) {
res.writeHead(403, { "Content-Type": "application/json" });
res.end(JSON.stringify({ error: "Forbidden" }));
} else {
res.writeHead(200, { "Content-Type": "application/json" });
res.end(JSON.stringify({ message: "Hello world" }));
}
});
server.listen(8000);
A Node.js server using a Shield rule.
Our cloud API is now deployed on Fly.io as well as AWS. The latest version of the SDK detects if you're running on Fly and will direct API calls to the API running there to minimize latency.
We're adding new regions based on demand, so let us know which regions you're using and we'll prioritize deployment.
We're adding more detailed verification options for developers where every request will be checked behind the scenes using published IP and reverse DNS data for common bots.
Arcjet security as code adapters for NestJS and Remix.
Support for Next.js 15 with performance improvements and full support for server actions.
Get the full posts by email every week.